Read full review of BookwormHub.com
One of the first function you’ll be introduced to when learning Python is the print() function. This article will cover how printing in Python works from the basics along with a few tricks you’d be interested to use in your projects. This function is commonly used in conjunction with python input techniques, and this article is the best guide to string formatting in python.The Basics of Print in Python
Given a string in python, the print function displays it in the console as an output. The function accepts not one, not two, but as many strings as you like to print in the console. Let’s work on an example: the task is to print “Hello world”. There are two ways to achieve this—either print one string “Hello world”, or two strings at the same time—“Hello” and “world”.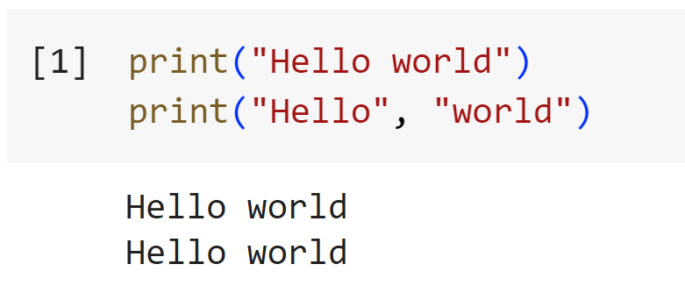
Syntax and Parameters
To understand the print function basics, below is the syntax in python of the print function. It consists of 4 optional parameters—sep, end, file, and flush:print(*objects, sep=' ', end='\n', file=None, flush=False)The *objects parameter takes in a string (or multiple strings) as we discussed above and is the primary parameter for printing strings. The optional sep parameter specifies the string that is used to separate each of the object strings passed (this is of course only relevant if we are printing multiple strings at once!). By default, the value of sep is simply a space character, which is why our output for print(“Hello”, “world”) above was “Hello world” (instead of “Helloworld”). The end parameter specifies the string to print at the end of the print statement. By default, the end parameter is ‘\n’ which is the line-break character. The file parameter specifies a file object to write the print output to. By default, the file parameter (if None) is set to sys.stdout (i.e., standard system output—which is your console!). The flush parameter is a rather advanced topic for most, however to simply put—output to console or a file is normally buffered (i.e., not immediately written) for performance reasons. This feature may be toggled by specifying the flush parameter.
Customizing Print Output
To customize the output of print function, we will focus on the first 3 parameters—*objects, sep, and end. Setting sep to ‘\n’ (i.e., line break) lets us print a series of strings in different lines—all using just one print function-call. Below is an example of using the custom separator (‘\n’).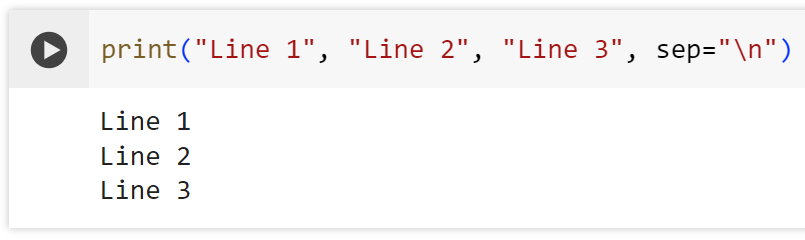

Working with Strings and Objects
Python’s print function goes a step further. The function displays not just strings, but numbers and even complex objects (like dictionaries and lists!). This is something that isn’t readily possible using string concatenation, but definitely something that the print function can undertake.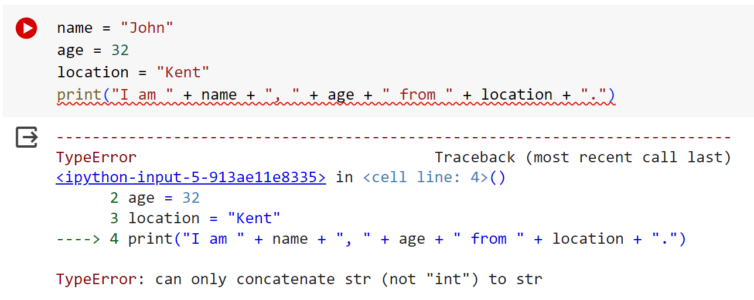
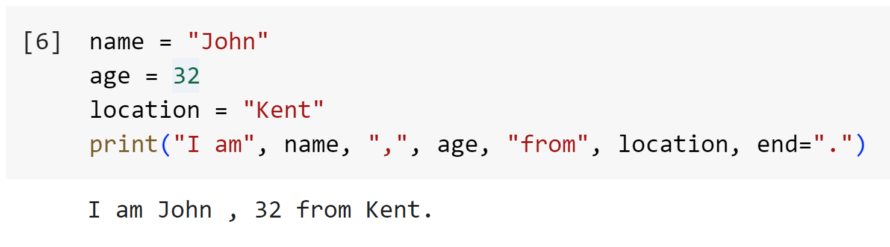
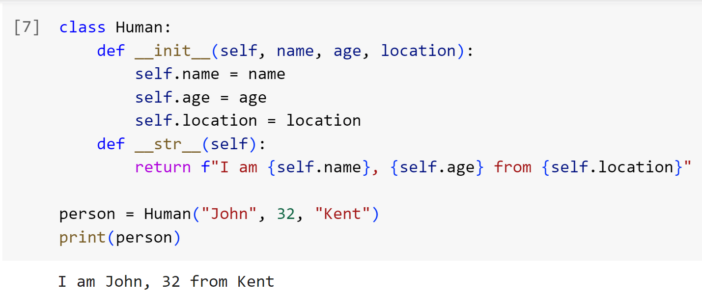
Practical Examples of Print Function
As we’ve discovered from prior examples, the versatility of the print function allows us to print strings in a much more readable manner. This is especially useful when printing data for logging. Consider the previous scenario where we have a Human class representing a person with the attributes—name, age, and location. Using the __str__ object overload, we define a string representation for class attributes and print log messages for our game server this way: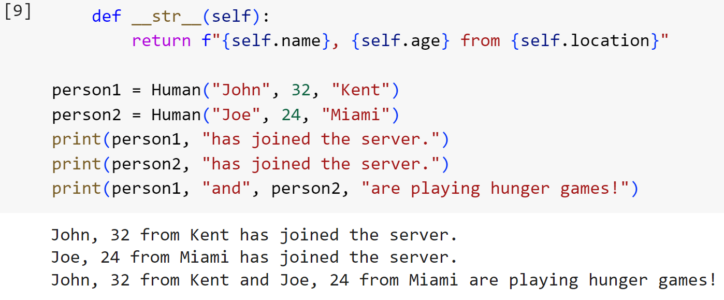
Advanced Techniques with Print
An often-overlooked parameter of the print function is the file parameter—a powerful feature that extends the utility of print beyond just the console. This parameter allows writing nicely formatted strings to a file, which is yet another bonus for logging mechanisms.Redirecting Output
The simplest use of the file parameter is to redirect output from the console to a file. To do this, you first need a file object that you can obtain by using Python’s open function.

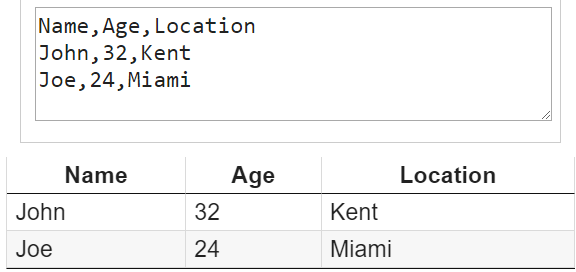